.Net Core Identity 做 Authorize
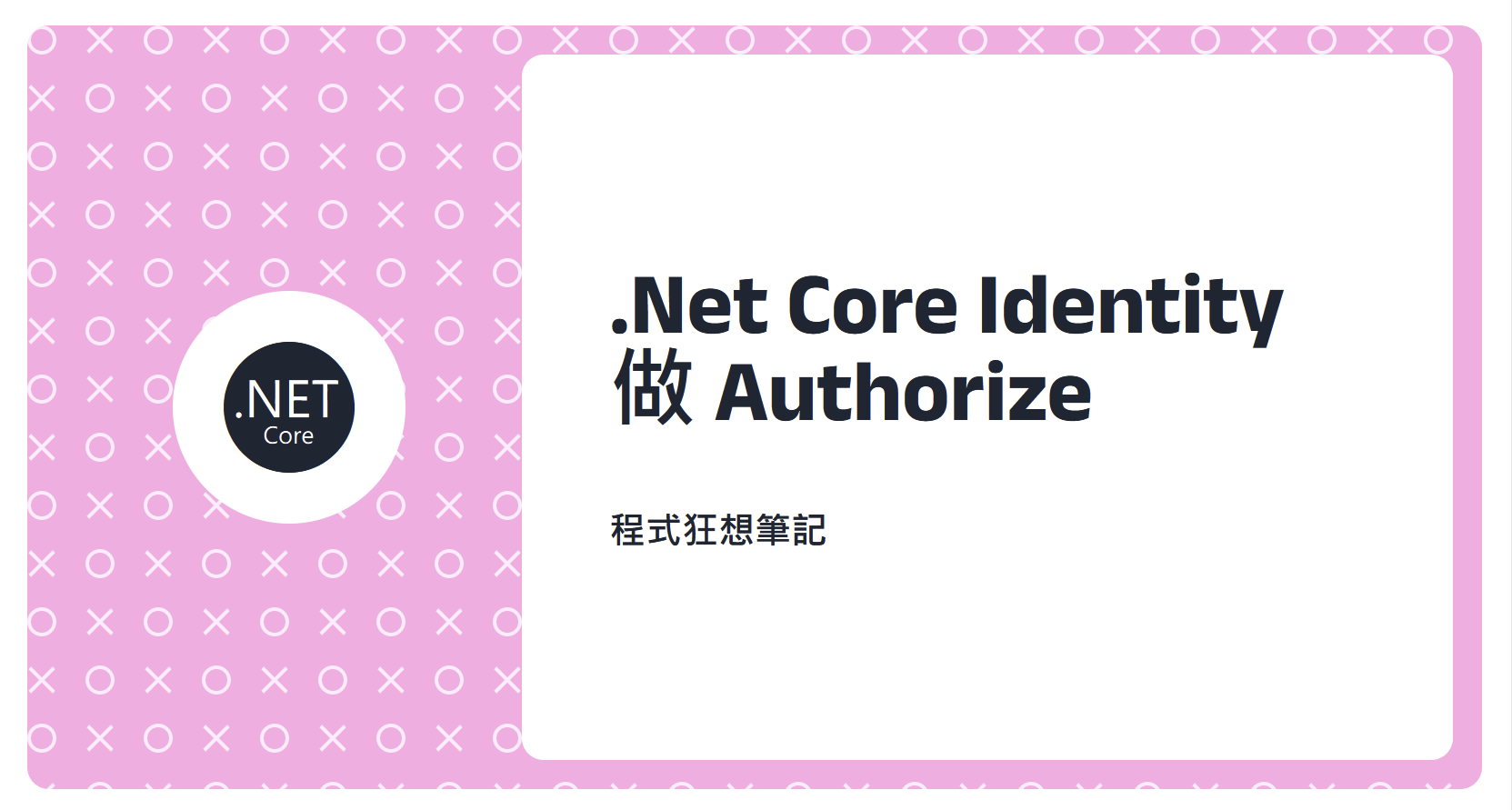
驗證與授權是很常用在帳號登入權限判斷,驗證(Authientication)
和授權(Authorization)
要先瞭解這兩個名詞才能順暢了解範例在做什麼。
驗證與授權關係
驗證(Authientication)
判斷是否有登入帳號資訊
授權(Authorization)
判斷使用者是否能有網頁資源(Resource)。不是只驗證使用者帳號,重點是否有權限進入頁面。
以上簡單用自己了解說明。詳細可Google查詢。
了解這兩個名詞在實作接下來內容才可能比較清楚
Authorize 屬性
GIT: AccountManage 加入授權設定 · malagege/NetCoreAuthSample@bc5bb3f
Controller 用上[Authorize]
就可以判斷使用者能否取得資源。這邊可以用來判斷使用者是否登入過帳號,這邊可以針對角色做調整,後續文章會在整理。
可在class上面加上[Authorize]
,每個 method 都會做授權判斷。也可以獨立在 method 做。
多角色授權注意事項
下面為 Admin或 User 角色可以授權此類別。
|
|
下面為Admin和User兩者角色符合才能授權此類別。
|
|
使用上需要注意。
全局授權驗證
Middleware 設定做確認授權動作
參考:ASP.NET Core中以原則為基礎的授權 | Microsoft Docs
|
|
filter 註冊
|
|
參考:Asp.Net Core Authorize你不知道的那些事(源码解读) - Jlion - 博客园
推薦好奇源碼怎麼做到,可以看裡面內容
AccessDenied 訪問頁面
GIT: AccessDenied 拒絕訪問功能 · malagege/NetCoreAuthSample@066d9d2
我們暫時 Authorize 把 Roles 加上隨便一個角色,如下方程式碼。
|
|
開啟http://localhost:50000/admin/rolelist
會倒到http://localhost:50000/Account/AccessDenied?ReturnUrl=%2Fadmin%2Frolelist
。這邊可以看到得到404結果。
這邊我們要寫死一個類別方法,在AuthSample\Controllers\AccountController.cs
有面新增一個AccessDenied
方法,在AuthSample\Views\Account\AccessDenied.cshtml
顯示錯誤訊息。
|
|
AuthSample\Views\Account\AccessDenied.cshtml
|
|
API 錯誤回應
這個用在 WebAPI感決怪怪的。我在Authentication In An ASP.NET Core API - Part 1: Identity, Access Denied | Pioneer Code有看到解決方式
|
|
其他參考:[ASP.NET Core] 啟用 UseAuthentication 後,讓MVC及API驗證失敗時有不同的行為 | Ian Chen - 點部落
但我看很多 API 都純後端可能沒加 CookieAuthenticationDefaultsm 驗證,所以可有做這個驗證。
.NET Core 2.0 Cookie Events OnRedirectToLogin - Stack Overflow
AllowAnonymous 屬性
GIT: AccountManage 加入授權設定 · malagege/NetCoreAuthSample@bc5bb3f
做 Authorize 動作的class有些方法需排除驗證授權,這邊需要加上[AllowAnonymous]
。
登入失敗會自動導入登入頁
GIT: 登入導向頁面 · malagege/NetCoreAuthSample@62198ab
http://localhost:50000/AccountManage/
像是會自動導回http://localhost:50000/Account/Login?ReturnUrl=%2FAccountManage%2F
。
所以login
需要特別做ReturnUrl
處理。
相關文章
Asp.Net Core Authorize你不知道的那些事(源码解读) - Jlion - 博客园
[Day14] ASP.NET Core 2 系列 - Filters - iT 邦幫忙::一起幫忙解決難題,拯救 IT 人的一天
ASP.NET Core中如何定制AuthorizeAttribute 備份圖
自訂 AuthorizationMiddleware 的行為 | Microsoft Docs